PGW SDK for React Native
The 2C2P Payment Gateway (PGW) SDK For React Native simplified integration solution for flutter platform with 2C2P Payment Gateway (PGW) SDK, 2C2P Payment Gateway (PGW) UI SDK and 2C2P Payment Gateway (PGW) SDK Helper.
React Native without framework
Step 1: Add following dependencies to your package.json file:
npm install @2c2p/[email protected]
yarn add @2c2p/[email protected]
Step 2: Import react native classes:
import RTNPGW, {
APIEnvironment,
APIResponseCode,
ApplePayPaymentResponseCode,
DeepLinkPaymentResponseCode,
GooglePayAPIEnvironment,
GooglePayPaymentResponseCode,
InstallmentInterestTypeCode,
PaymentCustomDataCode,
PaymentChannelCode,
PaymentInputCode,
PaymentNotificationPlatformCode,
PaymentUIResponseCode,
QRTypeCode,
ZaloPayAPIEnvironment,
ZaloPayPaymentResponseCode
} from '@2c2p/pgw-sdk-react-native';
- Android:
Step 3.1: Update your Android project build.gradle file.
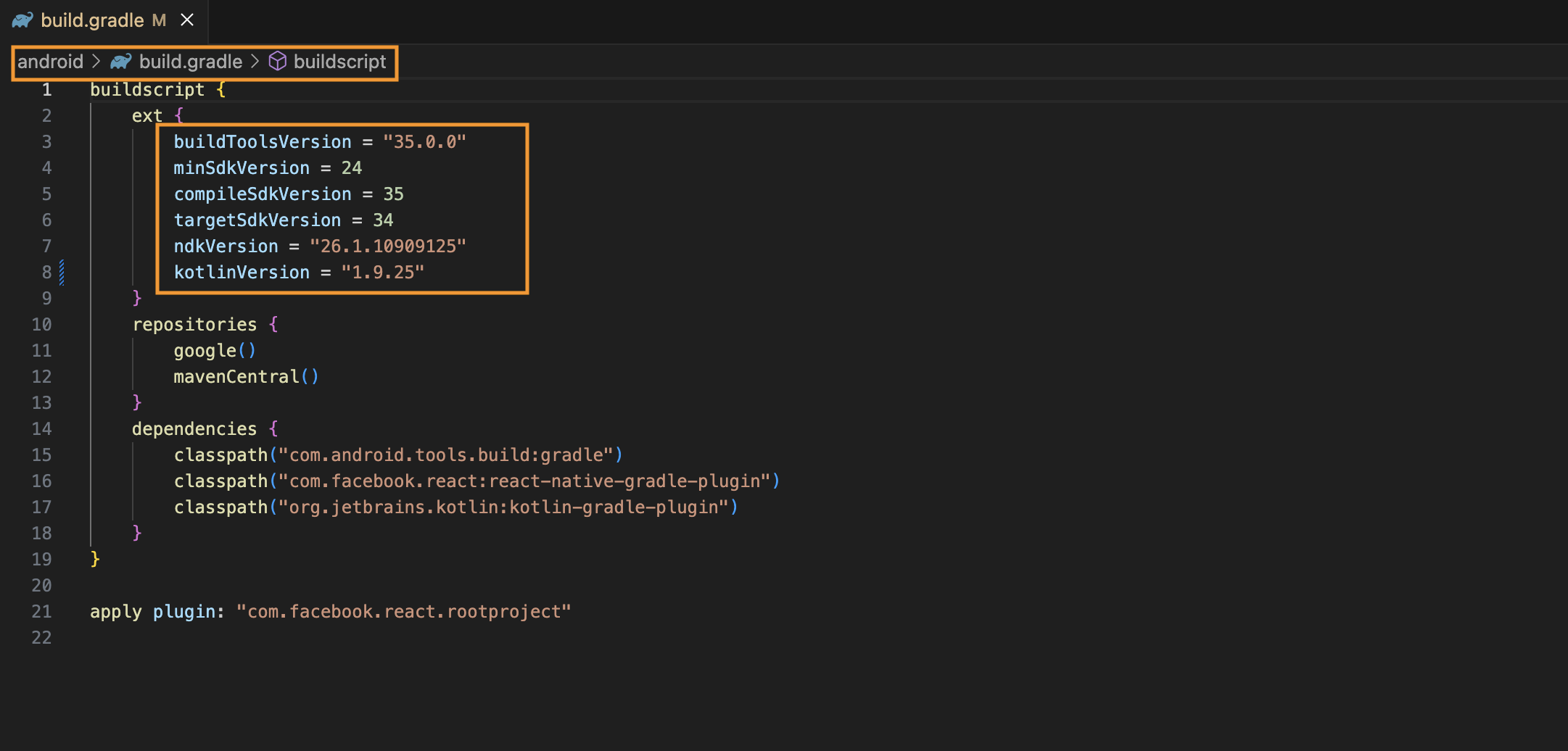
Step 3.2: Update build architectures in Android project gradle.properties file.
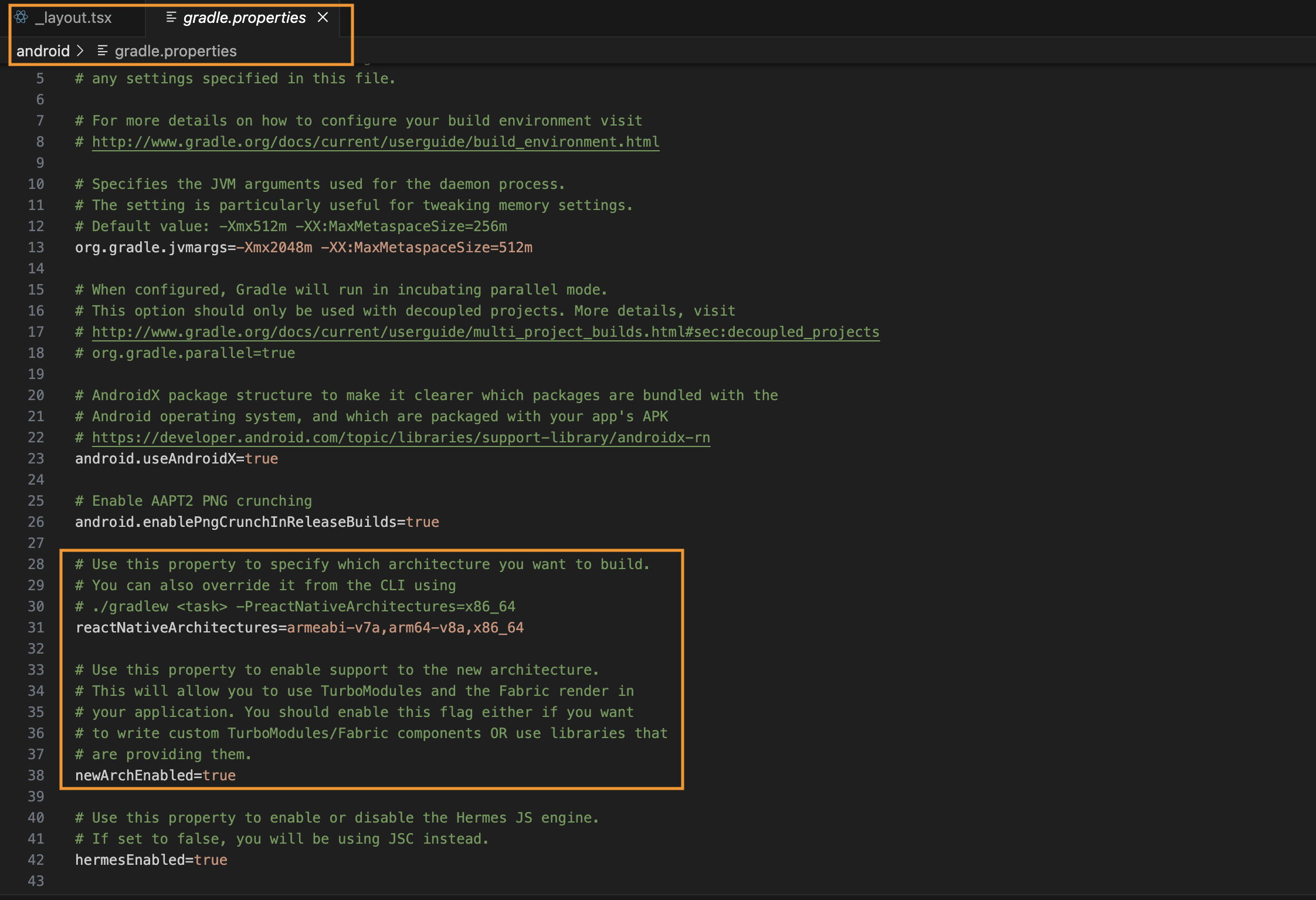
Build architecture: reactNativeArchitectures=armeabi-v7a,arm64-v8a,x86_64
New architecture: newArchEnabled=true
Old architecture: newArchEnabled=false
Step 3.3: Add into your Android project proguard file.
#React Native
-keep class com.facebook.react.** { *; }
-keepnames class com.facebook.react.* { *; }
-keepnames interface com.facebook.react.* { *; }
#PGWReactNative
-keep class com.ccpp.pgw.sdk.reactnative.** { *; }
-keepnames class com.ccpp.pgw.sdk.reactnative.* { *; }
-keepnames interface com.ccpp.pgw.sdk.reactnative.* { *; }
#React Native Plugins
-keep class com.swmansion.rnscreens.** { *; }
-keepnames class com.swmansion.rnscreens.* { *; }
-keepnames interface com.swmansion.rnscreens.* { *; }
- iOS:
Step 4.1: Execute download dependencies frameworks to iOS project.
npm explore @2c2p/pgw-sdk-react-native -- npm run ios-frameworks
cd node_modules/@2c2p/pgw-sdk-react-native && yarn run ios-frameworks && cd ../../..
Step 4.2: Go to iOS folder.
cd ios
Step 4.3: Execute pod bundle install.
New architecture: RCT_NEW_ARCH_ENABLED=1 bundle exec pod install
Old architecture: RCT_NEW_ARCH_ENABLED=0 bundle exec pod install
Step 4.4: Add deep link payment handle into your iOS Runner.xcodeproj. Reference: [Here]
Step 4.5: Add Apple Pay handle into your iOS Runner.xcodeproj. Reference: [Here]
Step 4.6: Add required info to your project Info.plist for Payment UI feature. Reference: [Here]
Expo framework
Step 1: Add following dependencies to your package.json file:
npx expo install @expo/config-plugins expo-build-properties @2c2p/[email protected]
Step 2: Import react native classes:
import RTNPGW, {
APIEnvironment,
APIResponseCode,
ApplePayPaymentResponseCode,
DeepLinkPaymentResponseCode,
GooglePayAPIEnvironment,
GooglePayPaymentResponseCode,
InstallmentInterestTypeCode,
PaymentCustomDataCode,
PaymentChannelCode,
PaymentInputCode,
PaymentNotificationPlatformCode,
PaymentUIResponseCode,
QRTypeCode,
ZaloPayAPIEnvironment,
ZaloPayPaymentResponseCode
} from '@2c2p/pgw-sdk-react-native';
- Android:
Step 3.1: Update your Android project build.gradle file and proguard file by autogenerate code. Reference: [app.json]
{
"expo": {
...
"android": {
...
"package": "com.ccpp.pgw.sdk.reactnative.demo"
},
"plugins": [
...
[
"expo-build-properties",
{
"android": {
"kotlinVersion": "1.9.25", //Only for Expo SDK <= 52
"enableProguardInReleaseBuilds": true,
"extraProguardRules": "#React Native\n-keep class com.facebook.react.** { *; }\n-keepnames class com.facebook.react.* { *; }\n-keepnames interface com.facebook.react.* { *; }\n\n#PGWReactNative\n-keep class com.ccpp.pgw.sdk.reactnative.** { *; }\n-keepnames class com.ccpp.pgw.sdk.reactnative.* { *; }\n-keepnames interface com.ccpp.pgw.sdk.reactnative.* { *; }\n\n#React Native Plugins\n-keep class com.swmansion.rnscreens.** { *; }\n-keepnames class com.swmansion.rnscreens.* { *; }\n-keepnames interface com.swmansion.rnscreens.* { *; }"
}
}
]
],
...
}
}
Step 3.2: Update build architectures in Android project gradle.properties file by autogenerate code with plugins. Reference: [app.json] / [plugins]
{
"expo": {
...
"newArchEnabled": true,
"plugins": [
...
"./plugins/withAndroidABIFilters"
],
...
}
}
- iOS:
Step 4.1: Execute download dependencies frameworks to iOS project.
npm explore @2c2p/pgw-sdk-react-native -- npm run ios-frameworks
cd node_modules/@2c2p/pgw-sdk-react-native && yarn run ios-frameworks && cd ../../..
Step 4.2: Add deep link payment handle into your iOS Runner.xcodeproj by autogenerate code with plugins. Reference: [app.json] / [plugins]
{
"expo": {
...
"newArchEnabled": true,
"ios": {
"bundleIdentifier": "com.ccpp.pgw.sdk.reactnative.demo",
"infoPlist": {
"LSApplicationQueriesSchemes": [
"zalo",
"zalopay",
"zalopay.api.v2",
"scbeasy",
"momo",
"line"
],
...
"CFBundleURLTypes": [
{
"CFBundleTypeRole": "Editor",
"CFBundleURLName": "2c2p",
"CFBundleURLSchemes": [
"pgwcom.ccpp.pgw.sdk.reactnative.demo"
]
}
]
},
...
},
"plugins": [
"./plugins/withIOSURLHandlerSwift" //For Expo SDK <= 52 use withIOSURLHandler, Expo SDK >= 53 use withIOSURLHandlerSwift
],
...
}
}
Step 4.3: Add Apple Pay handle into your iOS Runner.xcodeproj by autogenerate code. Reference: [app.json]
{
"expo": {
...
"newArchEnabled": true,
"ios": {
"bundleIdentifier": "com.ccpp.pgw.sdk.reactnative.demo",
...
"entitlements": {
"com.apple.developer.in-app-payments": [
"merchant.sg.702702000000000"
]
},
...
},
...
}
}
Step 4.4: Add required info to your project Info.plist for Payment UI feature by autogenerate code. Reference: [app.json]
{
"expo": {
...
"newArchEnabled": true,
"ios": {
"bundleIdentifier": "com.ccpp.pgw.sdk.reactnative.demo",
"infoPlist": {
...
"CADisableMinimumFrameDurationOnPhone": true,
"NSPhotoLibraryUsageDescription": "This app needs access to your photo library to save QR code images.",
...
}
},
...
}
}
Step 5: Run pre-build for iOS and android project.
npx expo prebuild --clean
Step 6: Verify all the app.json and plugin files configuration autogenerated code was applied to iOS and android project.
Step 7: Run iOS / Android app.
iOS: npx expo run:ios
Android: npx expo run:android
System Requirements
The SDK has been developed on following platform version:
Platform | Minimum Version |
---|---|
iOS | 12.4+ |
Android | 21+ (OS 5.0) |
React Native | 0.70.0+ |
Changelog
Version | Description | Resource |
---|---|---|
4.0.3 |
| |
4.0.2 |
| |
4.0.1 |
| |
4.0.0 |
|
Updated 2 months ago